Blazor and React, being two powerful tools for building SPAs, take a different approach. While React is a very popular JavaScript library from Facebook that has gained extensive use due to flexibility and a vast ecosystem, Blazor is a kind of Microsoft framework that enables a developer to write web applications using C# and Razor syntax, which makes it more suitable for .NET environments.
We will compare their performance, scalability, ease of use, and best use cases to help you decide on the right framework for your project. Check out our Q&A section where we answer common questions about Blazor as an alternative to React, Vue, and Angular. If you're unsure which technology fits your needs, our team is here to help!
What is Blazor?
What is Blazor in .NET? Blazor is an open-source web development framework created by Microsoft that enables developers to create interactive web applications using C#, HTML, and CSS, which means no more JavaScript. It runs on WebAssembly (WASM), so the application will execute directly in the browser, not requiring additional plugins or third-party dependencies.
One of the benefits of Blazor is that it interoperates seamlessly with JavaScript so that .NET methods can call JavaScript functions, and vice versa. Moreover, Blazor allows client-side and server-side to be united with C# and therefore makes sharing code, libraries, and components in the application much easier. It will mean that it has become a powerful tool in the generation of modern, scalable, and platform-independent SPAs.
Features of Blazor
Blazor is an open-source framework developed by Microsoft, and it helps simplify web development with C#, HTML, and CSS. It's a modern and efficient way to build interactive web applications. Some of its stand-out features are as follows:
1. Two Hosting Models: Blazor Server & Blazor WebAssembly
Blazor has two main hosting models:
- Blazor Server: The application runs on an ASP.NET Core server, and UI updates, JavaScript calls, and event handling are communicated over SignalR. This model provides full.NET API compatibility and faster load times but may have higher latency.
- Blazor WebAssembly (WASM): These applications run natively in the browser by downloading all needed files, including the .NET runtime, to the client. That makes it suitable for static site hosting with little server dependency and also supports offline applications.
2. Complete HTML and CSS Support
Blazor renders UI components as vanilla HTML and CSS and, therefore, plays nice with most CSS frameworks, including Tailwind and Bootstrap, but also supports:
- CSS isolation, ensuring styles don’t interfere across components.
- Preprocessors like SASS for advanced styling.
- Built-in form components to simplify form generation and validation.
3. Server-Side Pre-Rendering
Blazor improves page load speeds by rendering content on the server before sending static HTML to the client. This enhances:
- SEO optimization by reducing load delays.
- The user experience by displaying UI elements even before the full app is downloaded.
4. Virtualization for Best Rendering
Virtualization lets Blazor render only the visible UI elements, thereby significantly improving performance when dealing with large datasets. It does not render thousands of items at a time but rather loads the visible portion dynamically. Example:
Without Virtualization
@foreach (var item in items)
{
<tr>
<td>@item.Idtd>
<td>@item.Nametd>
tr>
}
With Virtualization
<Virtualize Items="items" Context="item">
<tr>
<td>@item.Idtd>
<td>@item.Nametd>
tr>
Virtualize>
5. Hot Reload for Rapid Development
. NET 6 has introduced Hot Reload, which enables developers to see changes immediately in the browser by altering code without requiring the full application reload, thus increasing productivity.
6. Lazy Loading for Performance
Lazy loading is supported by Blazor WebAssembly, which loads up only the required libraries and resources. This makes initial loads faster, as non-essential resources are deferred.
Example:
<ItemGroup>
<BlazorWebAssemblyLazyLoad Include="MyLibrary.dll" />
ItemGroup>
7. gRPC-Web Integration for High-Performance Communication
Blazor supports gRPC-Web as a high-performance communication Blazor Framework, thus ensuring faster communication between the client and the server-side apps with increased efficiency and security.
Blazor Pros and Cons
Blazor is a web framework that lets developers build interactive web applications using C# and .NET rather than JavaScript. It has two main hosting models: Blazor WebAssembly (WASM) and Blazor Server, with pros and cons for each.
Blazor WebAssembly (WASM)
Pros
- Runs.NET applications directly in the browser, so there is no need for JavaScript frameworks.
- Supports offline functionality, making it perfect for progressive web apps (PWAs).
- Interoperability with JavaScript, and the ability to reuse existing JS libraries.
- Most modern browsers do not require extra plugins or compilers.
Cons
- Long load times on slow networks since the whole.NET runtime needs to be downloaded.
- Compared to other.NET applications, fewer debugging tools are available.
- Not supported on thin clients. A sufficiently powerful client side is required
Blazor Server
Pros
- Loads faster than Blazor WASM since only UI updates need to be sent to the client.
- Smaller app size, with better performance and bandwidth usage.
- Supports all browsers, even those that don't have WebAssembly support.
- Runs on the full.NET Core runtime, giving access to the full range of.NET features.
Cons
- Scalability issues since it relies on a persistent SignalR connection for real-time UI updates.
- Offline support is not available since it needs a constant server connection.
- High latency problems can cause a bad user experience in slow network environments.
What is React?
React is the JavaScript library for building user interfaces and reusable UI components flexibly and dynamically. Issued by Facebook in 2013, React has become one of the most widely used UI libraries for creating intuitive and efficient user interfaces. Companies such as PayPal, Dropbox, Twitter, Netflix, and Walmart make use of React for their applications.
Before the release of React, developers were using frameworks such as Angular and Vue, which often demanded significant code rewrites. The introduction of React brought a component-based architecture, where developers could reuse code efficiently, streamline the .NET Development Services process, and simplify the maintenance of SPAs.
Features of React
React is a strong JavaScript library, primarily used to develop dynamic and fast user interfaces. It offers numerous React Features that ensure faster development time, enhanced React performance, and a simplified user interface management process. Some of the key React Features include the following.
1. Extension of JavaScript Syntax (JSX)
This is a combination of JavaScript and HTML, allowing the developer to write HTML-like syntax directly in JavaScript. That makes the code more readable and maintainable with easier debugging capabilities. JSX also renders UI components faster than in traditional JavaScript.
Example
const name = 'JSX Sample';
const element = <h1>This is a {name}h1>;
Since JSX is not actual JavaScript, Babel has to compile it to browser-compatible JavaScript.
2. Virtual DOM
React uses a Virtual DOM to make the React performance better by avoiding unnecessary updates in the real DOM. Instead of updating the whole page when there is a change, React:
- Makes a virtual copy of the DOM in memory.
- Compare it with the previous version (diffing).
- Updates only the changed elements in the real DOM (reconciliation).
This optimization makes the app run much faster and more efficiently than traditional DOM manipulation.
3. One-Way Data Binding
React follows a unidirectional data flow, meaning that data moves in a single direction, from parent components to child components. This approach:
- Ensures better control over data within the application.
- Prevents unexpected side effects.
- Makes debugging and tracking data changes easier.
Although child components cannot modify parent data directly, they can send requests to update states, ensuring a modular and predictable application structure.
4. Component-Based Architecture
React breaks down the UI into reusable components, each with its properties and functionality. This approach:
- Makes code management and debugging easier.
- Promotes code reusability, thus saving time in development.
- Enhances modularity, which makes complex UI structures easier to handle.
Key Features of the Component
- Nesting: Components can contain several sub-components.
- Render Method: Specifies how UI elements will appear in the DOM.
- Props (Properties): Allows data to be passed from parent to child components.
5. Extension Support
React supports many extensions that enhance its functionality, including the following:
- Redux & Flux: State management tools for complex data.
- React Native: Supports mobile app development with React.
- Next.js: Server-side rendering and static site generation.
Pros and Cons of React
Pros
- Reusability of Components: It is based on component-based architecture wherein the developers write the reusable components of the User Interface and reduce redundancy in their development to increase efficiency.
- Virtual DOM for Improved Performance: The updated parts of the real DOM are only done in React, making rendering faster and application performance better.
- It can be said to have declarative syntax: That is, what the UI looks like, describing it makes for easier reading and debugging as well as easier maintainability of code.
- Extensive Community Support: The large active community offers significant resources, tutorial material, libraries, and many third-party libraries and tools designed to solve real-world problems.
- One-way Data Binding: the unidirectional flow of React guarantees more controls over data that will lead to easier debugging that makes it apt for the most massive applications of all.
Cons
- JSX Complexity: JSX, React’s syntax extension, can be challenging for beginners unfamiliar with JavaScript and HTML mixing, adding a learning curve.
- Steep Learning Curve: While React is powerful, mastering concepts like state management, hooks, and context API can be difficult for new developers.
- Complex Tooling Setup: Setting up a React development environment requires configuring Webpack, Babel, and other tools, which can be time-consuming and complicated for beginners.
- Inconsistent Documentation: Some developers feel that React's documentation is not clear enough, especially for advanced topics, which makes it difficult to understand complex concepts without additional resources.
- SEO Issues: Applications built with React that are client-side rendered may have SEO issues because search engines will have a hard time crawling and indexing dynamic content. Solutions like Next.js can help mitigate this problem.
Blazor vs React: Differences
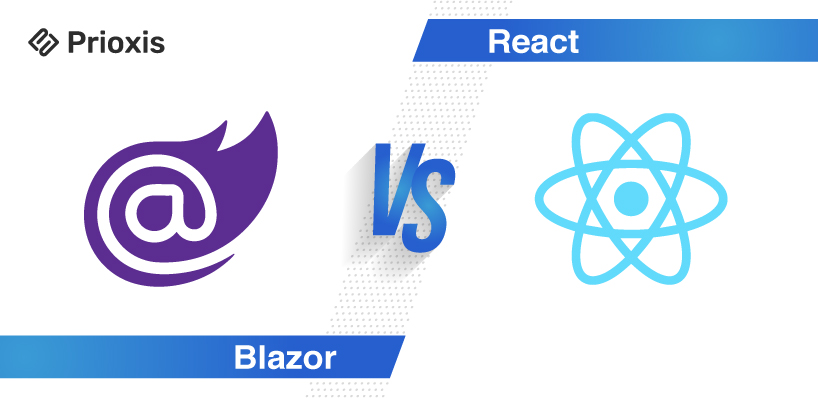
1: Framework vs. Library
- Blazor: A full-fledged framework developed by Microsoft for building interactive web applications using C# and.NET.
- React: A JavaScript library maintained by Facebook, primarily focused on building user interfaces.
2: Primary Language
- Blazor: Uses C# and .NET for both client-side and server-side development.
- React: Primarily uses JavaScript (or TypeScript) to create user interfaces.
3: Component Model
- Blazor: It follows a component-based model, which encapsulates both the UI and its logic within reusable components.
- React: It uses a virtual DOM to efficiently manage and render UI components, thereby reducing direct interactions with the actual DOM.
4: Developer Familiarity
- Blazor: It is easier for developers who have experience with C# and.NET technologies.
- React: It is more accessible for those familiar with JavaScript, but it has a steeper learning curve due to its ecosystem and concepts.
5: Deployment
- Blazor: It can be deployed to any platform. When combined with ASP.NET Core, it's specifically targeted toward full-stack solutions.
- React: It can be deployed on any platform that supports static file deployment. Netlify and Vercel are often used.
6: Use Cases
- Blazor: Used for data-intensive applications where there is heavy use of business logic processing on the server side.
- React: It is suited to complex user interface-intensive applications
with fast client-side rendering.
7: Development Environment
- Blazor: Works with Visual Studio, giving users vast debugging and profiling capabilities.
- React: Uses many third-party tools and libraries, which can be flexible but need extra setup.
8: Performance
- Blazor: Server-side Blazor is likely to have increased latency due to round trips between the server and client. Client-side Blazor is more low-latency but slower because it loads the .NET runtime.
- React: Generally faster for DOM manipulations due to its virtual DOM, which means that the UI updates are very efficient.
9: Ecosystem
- Blazor: Its ecosystem is growing, but it is not as large as that of React.
- React: It has a huge ecosystem of third-party libraries, packages, and extensions that can add functionality and speed up development.
Which One Would You Choose?
Selecting React vs Blazor depends primarily on the criteria, which could include the following considerations:
1: Developer Proficiency
- Blazor: Best to apply for team working with experiences from C # or .Net.
- React: Best with an experienced developer for JavaScript and TypeScript in most cases.
2: Project Requirements
- Blazor: Efficiently handles complex backend logic.
- React: Optimized for high-performance client-side rendering.
3: Development Environment
- Blazor: Ideal for developers who use Visual Studio.
- React: More flexible; accommodates a vast array of tools and configurations.
4: Long-term Consideration
- Blazor: Aligns with organizations that are committed to the Microsoft stack.
- React: Community support is really strong, and industry application is vast.
Use Blazor for data-centric applications within a.NET environment, or use React for dynamic, user-focused interfaces. Your choice will depend on your project's needs and the strengths of your team.
Final Words
In the Blazor vs. React discussion, you should know that there is no silver bullet. Both frameworks are incredibly well made and mostly depend on your project's different requirements.
In case you want to have efficient and highly performing solutions, Prioxis provides the best server hosting. You can deploy your meticulously developed applications in our robust infrastructure, feeling confident that you won't compromise on the quality.
With Prioxis, you will have a stable base for your project, freeing you to do what you are best at: creating great web applications.